Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial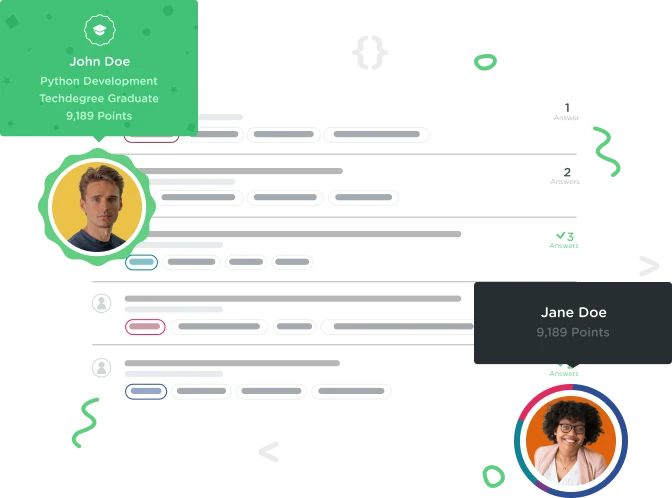

Keon Park
11,637 PointsDon't know the problem with my minimax algorithm
I am trying to write a minimax algorithm for my tictactoe game but it just returns random moves. Here is my code:
def minimax_score(board, player): # player will be X for simplicity
original_player = player
empty_spots = []
other_player = None
if player == 'X':
other_player = 'O'
elif player == 'O':
other_player = 'X'
for y in range(3): # find all open spots on board
for x in range(3):
if board[y][x] == None:
empty_spots.append([y, x])
if ttt.get_winner(board) == player:
return 10
elif ttt.get_winner(board) == other_player:
return -10
elif ttt.get_winner(board) == None and empty_spots == []:
return 0
scores = []
moves = []
for y in range(3): # find all open spots on board
for x in range(3):
if board[y][x] == None:
moves.append([y, x])
for move in moves: # if board state is not terminal
board[move[0]][move[1]] = player
if player == 'X':
player = 'O'
elif player == 'O':
player = 'X'
scores.append(minimax_score(board, player))
board[move[0]][move[1]] = None
player = original_player
if player == 'X':
return max(scores)
elif player == 'O':
return min(scores)
def minimax_ai(board, player):
best_move = None
best_score = None
moves = []
for y in range(3): # find all open spots on board
for x in range(3):
if board[y][x] == None:
moves.append([y, x])
for move in moves:
new_board = copy.deepcopy(board)
new_board[move[0]][move[1]] = player
if player == 'X':
player = 'O'
elif player == 'O':
player = 'X'
score = minimax_score(new_board, player)
new_board[move[0]][move[1]] = None
if best_score is None or score > best_score:
best_move = [move[1], move[0]]
best_score = score
return best_move
minimax_score gives the score for the current state of the tictactoe board. I have no clue on how to go about this problem. I apologize for how bad the code looks, I am planning on fixing it later. I wrote this without much thought since it has not been long since I started coding.
boi
14,241 Pointsboi
14,241 PointsCould you describe the working principle of your code? like, which function should be run first, and what inputs should be given to each function, what results do you get in the REPL and what are the expected results you want? Show me in code format. I'll make sure to try to solve it.