Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial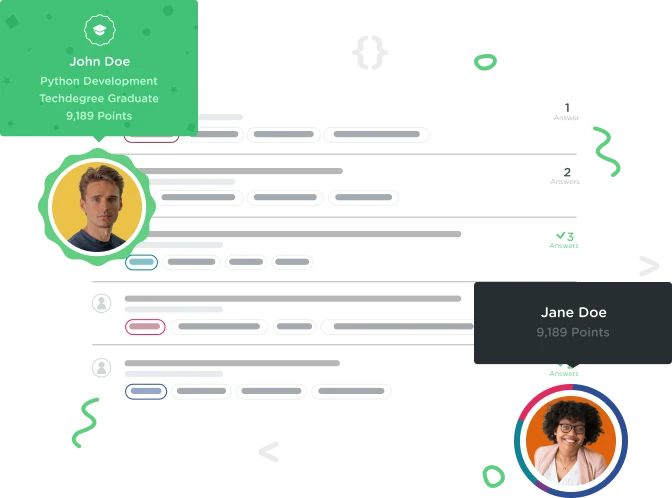

zach poll
1,087 PointsAny idea as to why my value for answering a question correctly is not adding up?
/*
- Store correct answers
- When quiz begins, no answers are correct */ let correct = 0 ;
// 2. Store the rank of a player let rank = 'iron';
// 3. Select the <main> HTML element const main = document.querySelector('main');
/*
- Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers */ const answer1 = prompt('Is the sun a star?' ); if ( answer1.toUpperCase() === 'yes' ) { correct += 1; } const answer2 = prompt('Is grass green?'); if ( answer2.toUpperCase() === 'yes') { correct += 1; } const answer3 = prompt('Is the sky blue?'); if ( answer3.toUpperCase() === 'yes' ) { correct += 1; } const answer4 = prompt('What day is it today?'); if ( answer4.toUpperCase() === 'Monday' ) { correct += 1; } const answer5 = prompt('What color is my water bottle?'); if ( answer5.toUpperCase() === 'Black' ) { correct += 1; }
/*
- Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown */ if ( correct === 5 ) { rank = "Gold"; } else if ( correct >= 3 ) { rank = "silver"; } else if ( correct >= 2 ) { rank = "Bronze"; } else { rank = "None :("; }
// 6. Output results to the <main> element
main.innerHTML =
<h2>You got ${correct} out of 5 qustions correct.</h2>
<p>Crown earned: <strong>${rank}</strong></p>
;
1 Answer

jb30
44,805 Pointsanswer1.toUpperCase() === 'yes'
converts the value of answer1
to uppercase, then compares it to the string yes
. Since yes
is not uppercase, the comparison will never be true. Try changing the condition to answer1.toLowerCase() === 'yes'
or to answer1.toUpperCase() === 'YES'
.
For answer4
and answer5
, you might want to modify the strings on the right side of the comparison to either be entirely uppercase (and call .toUpperCase()
on the answer on the left side of the comparison) or entirely lowercase (and call .toLowerCase()
on the answer on the left side of the comparison).